最終更新日:
公開日:
レシピ
文字列
文字列をURIエンコード(エスケープ)・デコードする
URIに含まれる日本語をエンコード/デコードする方法について解説します。
この記事のポイント
- 記号を含めてエンコードするときはencodeURIComponentメソッドを使う
- encodeURIメソッドでエンコードしたらdecodeURIメソッドでデコードする
- encodeURIComponentメソッドでエンコードしたらdecodeURIComponentメソッドでデコードする
目次
URIをエンコードする
URIは記号や日本語を含むことがあり、SNSで共有するときなどにそのままでは使用できないことがあります。
そこで、encodeURIメソッドかencodeURIComponentメソッドを使うと、URIをエンコードして通常のテキストとして使用できるようにすることができます。
以下の例では、日本語を含むURIを取得してエンコードし、通常のテキストに変換している例です。
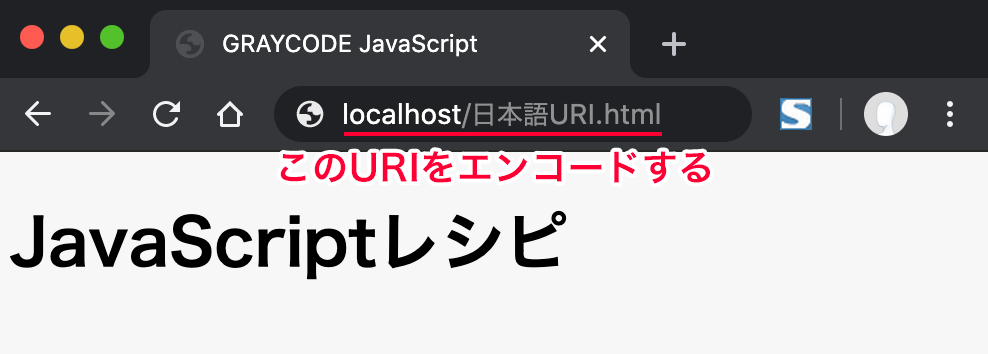
JS コード例
// URIを取得する
let uri = location.href; // http://localhost/日本語URI.html
console.log(encodeURI(uri));
// http://localhost/%25E6%2597%25A5%25E6%259C%25AC%25E8%25AA%259EURI.html
console.log(encodeURIComponent(uri));
// http%3A%2F%2Flocalhost%2F%25E6%2597%25A5%25E6%259C%25AC%25E8%25AA%259EURI.html
encodeURIメソッドとencodeURIComponentメソッドの違いは、次の記号も含めてエンコードするかどうかの挙動にあります。
JS コード例
let string1 = "/:;,?&=+$#@"; // 変換する記号
let string2 = "ABCDEF abcdef 1234567890"; // スペースは変換する
// string1の文字列をエンコードして出力
console.log(encodeURI(string1)); // /:;,?&=+$#@
console.log(encodeURIComponent(string1)); // %2F%3A%3B%2C%3F%26%3D%2B%24%23%40
// string2の文字列をエンコードして出力
console.log(encodeURI(string2)); // ABCDEF%20abcdef%201234567890
console.log(encodeURIComponent(string2)); // ABCDEF%20abcdef%201234567890
string2の文字列はエンコード結果は同じですが、string1の記号はencodeURIComponentメソッドのみエンコードされていることが分かります。
また、以下の記号はどちらのメソッドもエンコードしません。
JS コード例
let string3 = "-_.!~*'()";
console.log(encodeURI(string3)); // -_.!~*'()
console.log(encodeURIComponent(string3)); -_.!~*'()
URIをデコードする
エンコードしたURIをデコードしてエンコード前の状態に戻すには、decodeURIメソッドかdecodeURIComponentメソッドを使います。
JS コード例
// URIを取得する
let uri = location.href;
let encoded_uri1 = null;
let encoded_uri2 = null;
// URIをエンコード
encoded_uri1 = encodeURI(uri);
encoded_uri2 = encodeURIComponent(uri);
// URIをデコード
console.log( decodeURI(encoded_uri1));
console.log( decodeURIComponent(encoded_uri2));
// いずれも => http://js.localhost/%E6%97%A5%E6%9C%AC%E8%AA%9EURI.html
基本的にはエンコードの対象となる記号を等しくする必要があるため、encodeURIメソッドでエンコードした場合はdecodeURIメソッド、encodeURIComponentメソッドでエンコードした場合はdecodeURIComponentメソッドを使います。
エンコード実行後とデコード実行後を出力して変換した内容を確認すると、次のようになります。
まずはencodeURIメソッドとdecodeURIメソッドの組み合わせです。
JS コード例
let string1 = "/:;,?&=+$#@";
let string2 = "ABCDEF abcdef 1234567890";
let string3 = "-_.!~*'()";
let encoded_string1 = encodeURI(string1);
let encoded_string2 = encodeURI(string2);
let encoded_string3 = encodeURI(string3);
// エンコード実行後
console.log( encoded_string1); // /:;,?&=+$#@
console.log( encoded_string2); // ABCDEF%20abcdef%201234567890
console.log( encoded_string3); // -_.!~*'()
// デコード実行後
console.log( decodeURI(encoded_string1)); // /:;,?&=+$#@
console.log( decodeURI(encoded_string2)); // ABCDEF abcdef 1234567890
console.log( decodeURI(encoded_string3)); // -_.!~*'()
続いて、encodeURIComponentメソッドとdecodeURIComponentメソッドの組み合わせによる変換の過程を出力します。
JS コード例
let string1 = "/:;,?&=+$#@";
let string2 = "ABCDEF abcdef 1234567890";
let string3 = "-_.!~*'()";
let encoded_string1 = encodeURIComponent(string1);
let encoded_string2 = encodeURIComponent(string2);
let encoded_string3 = encodeURIComponent(string3);
// エンコード実行後
console.log( encoded_string1); // %2F%3A%3B%2C%3F%26%3D%2B%24%23%40
console.log( encoded_string2); // ABCDEF%20abcdef%201234567890
console.log( encoded_string3); // -_.!~*'()
// デコード実行後
console.log( decodeURIComponent(encoded_string1)); // /:;,?&=+$#@
console.log( decodeURIComponent(encoded_string2)); // ABCDEF abcdef 1234567890
console.log( decodeURIComponent(encoded_string3)); // -_.!~*'()
いずれも冒頭の変数とデコード後に出力した内容が等しくなるため、しっかりとエンコード&デコードされていることを確認できます。
こちらの記事は役に立ちましたか?
コメントありがとうございます!
運営の参考にさせていただきます。
ありがとうございます。
もしよろしければ、あわせてフィードバックや要望などをご入力ください。